- Thread Author
- #1
Well I got bored and made this mouse and thought it was kinda cool.
GIF of this:
Variables:
Methods:
Add to onStart():
Add to onStop():
GIF of this:
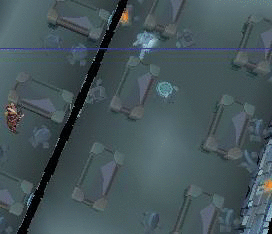
Variables:
Code:
public Color targetColor;
public Color currentColor;
long t = -1;
long timePerTick = 0;
private Runnable MOUSE_THREAD = new Runnable() {
@Override
public void run() {
while (true) {
if (t == -1 || t + timePerTick < System.currentTimeMillis()) {
t = System.currentTimeMillis();
int tr = targetColor.getRed(), tg = targetColor.getGreen(), tb = targetColor.getBlue();
int cr = currentColor.getRed(), cg = currentColor.getGreen(), cb = currentColor.getBlue();
int dr = tr - cr, dg = tg - cg, db = tb - cb;
currentColor = new Color(getColorFrom(dr, cr), getColorFrom(dg, cg), getColorFrom(db, cb));
if (currentColor.getRGB() == targetColor.getRGB()) {
targetColor = new Color(Random.nextInt(255), Random.nextInt(255), Random.nextInt(255));
}
}
}
}
};
Mouse.PathRenderer render = new PathRenderer() {
@Override
public void render(Graphics2D g) {
g.setColor(currentColor);
g.drawLine(Mouse.getPosition().x, 0, Mouse.getPosition().x, 3000);
g.drawLine(0, Mouse.getPosition().y, 3000, Mouse.getPosition().y);
}
};
public Thread thread = new Thread(MOUSE_THREAD);
Code:
private int getColorFrom(int d, int c) {
return c + (d / (Math.abs(d) == 0 ? 1 : Math.abs(d)));
}
Code:
targetColor = new Color(Random.nextInt(255), Random.nextInt(255), Random.nextInt(255));
currentColor = new Color(Random.nextInt(255), Random.nextInt(255), Random.nextInt(255));
Mouse.setPathRenderer(render);
thread.start();
Code:
thread.stop();
Last edited: