- Joined
- Nov 17, 2014
- Messages
- 4,906
- Thread Author
- #1
Since I recognized especially new bot authors struggle a little to find a good way to get into RuneMate's many pathing and traversing techniques, I've decided to finally write a basic introduction for all of them.
First, you need to find out which pathing system fits your needs in order to make your bot function properly. This depends on the distance your bot has to travel, and whether or not it needs to open doors or walk past certain buildings and stuff like that.
Option 1: WebPath
Option 4: PredefinedPath
Additional: ViewportPath
You also can combine those methods to ensure a good traversal, for example, let the bot generate a path with RegionPath, and nullcheck it in order to see if it is valid. If it is, you can let it walk, if not, attempt to build a WebPath, or BresenhamPath as a last resort.
First, you need to find out which pathing system fits your needs in order to make your bot function properly. This depends on the distance your bot has to travel, and whether or not it needs to open doors or walk past certain buildings and stuff like that.
WebPath requires a given web of vertices in order to build a path based on it. You can see the web as a big field of coordinates, which are linked to each other.
Coordinate A is linked to coordinate B if B is reachable from A. Using this system the web's pathbuilder can calculate a path along those links to your desired location.
The big advantage of it is that it can potentially go everywhere, as it supports obstacles like doors or shortcuts or even teleportations, assuming that your web supports those.
You can use RuneMate's default web (accessible through Traversal.getDefaultWeb()), or you can build your own using a web builder from the bot store (or write your own web builder). The default web covers the most common areas of the RuneScape surface, but is, afaik, not supporting shortcuts etc. yet.
To see if the default web manages to build a path to your desired location, you should test it yourself. If you want to use your own web, you need to load it from a file.
Use this pathing system if you need to travel a larger distance and/or if obstacles are in your way.
Option 2: RegionPathCoordinate A is linked to coordinate B if B is reachable from A. Using this system the web's pathbuilder can calculate a path along those links to your desired location.
The big advantage of it is that it can potentially go everywhere, as it supports obstacles like doors or shortcuts or even teleportations, assuming that your web supports those.
You can use RuneMate's default web (accessible through Traversal.getDefaultWeb()), or you can build your own using a web builder from the bot store (or write your own web builder). The default web covers the most common areas of the RuneScape surface, but is, afaik, not supporting shortcuts etc. yet.
To see if the default web manages to build a path to your desired location, you should test it yourself. If you want to use your own web, you need to load it from a file.
Use this pathing system if you need to travel a larger distance and/or if obstacles are in your way.
Code:
public class Walking extends Task {
private final Coordinate BANK_COORD = new Coordinate(x, y);
private Web web; // Custom web, gets loaded in constructor
public Walking() {
try {
web = FileWeb.fromByteArray(Resources.getAsByteArray("path/to/webfile")); // load the web with the Resources class, make sure to address this resource in your manifest
} catch (Exception e) {
e.printStackTrace();
web = null;
}
}
@Override
public void execute() {
//Use this if you use the default web:
final WebPath path = Traversal.getDefaultWeb().getPathBuilder().buildTo(BANK_COORD);
//Or this if you use your own web
final WebPath path = null;
if (web != null) { // Make sure the web got loaded properly
path = web.getPathBuilder().buildTo(BANK_COORD);
}
if (path != null) { // IMPORTANT: if the path should be null, the pathbuilder could not manage to build a path with the given web, so always nullcheck!
path.step();
}
}
@Override
public boolean validate() {
//validate
}
}
RegionPath acts similiar to WebPath, it builds a path based on a web, though in this case, the web represents the currently loaded region. Notice that just like WebPath, the pathbuilder can't generate any path to a coordinate which is not part of the web, so make sure your destination is located in your loaded region and is reachable (no doors shut or obstacles you have to climb over).
You may ask now, what is a region? A region is just a small area of the RuneScape world loaded into your RAM, once you enter another region, the game has to load the next region into the RAM, which causes the loading times during traversal you may know. RuneScape does this because it would be impossible to load the whole world at once.
So you can use this method if you are positive that your destination is within the loaded region and you just travel a short distance.
Option 3: BresenhamPathYou may ask now, what is a region? A region is just a small area of the RuneScape world loaded into your RAM, once you enter another region, the game has to load the next region into the RAM, which causes the loading times during traversal you may know. RuneScape does this because it would be impossible to load the whole world at once.
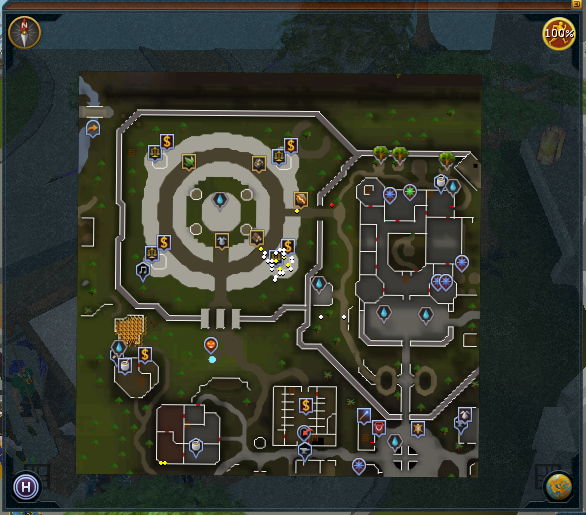
So you can use this method if you are positive that your destination is within the loaded region and you just travel a short distance.
Code:
public class Walking extends Task {
private final Coordinate bankCoord = new Coordinate(x, y);
@Override
public void execute() {
final RegionPath path = RegionPath.buildTo(bankCoord);
if (path != null) { // IMPORTANT: if the path should be null, the pathbuilder could not manage to build a path with the given region, so always nullcheck!
path.step();
}
}
@Override
public boolean validate() {
//validate
}
}
If you google up "Bresenham Path" you can guess very well what this does. It just draws a straight line from Coordinate A to Coordinate B, not giving a fuck whether it is even reachable or not. So pay attention if you use this method, even because most players won't travel in one accurate line, increasing the chance of a ban.
The advantage of this method is that it is more lightweight than building a path over and over, calculating multiple things etc.
Use this method only if the path you want to take is very short and hast little to no obstacles in between.
The advantage of this method is that it is more lightweight than building a path over and over, calculating multiple things etc.
Use this method only if the path you want to take is very short and hast little to no obstacles in between.
Code:
public class Walking extends Task {
private final Coordinate bankCoord = new Coordinate(x, y);
@Override
public void execute() {
final BresenhamPath path = BresenhamPath.buildTo(bankCoord);
if (path != null) { // Although BresenhamPath technically always builds a path, it is recommended to nullcheck rather than having the bot crash
path.step();
}
}
@Override
public boolean validate() {
//validate
}
}
Option 4: PredefinedPath
As the name says, PredefinedPath takes predefined coordinates in order to traverse along them. This method can be good if you need to take a very special route across the landscape in order to avoid getting attacked by monsters for example.
There are tools on the internet (which are a bit old though) which can help you generate an array of coordinates. Alternatively you can find out the coordinates the path should be ingame using the devkit.
There are tools on the internet (which are a bit old though) which can help you generate an array of coordinates. Alternatively you can find out the coordinates the path should be ingame using the devkit.
Code:
public class Walking extends Task {
private final Coordinate[] pathCoords = {new Coordinate(x, y), new Coordinate(x, y), new Coordinate(x, y)};
@Override
public void execute() {
PredefinedPath.create(pathCoords).step();
}
@Override
public boolean validate() {
//validate
}
}
Additional: ViewportPath
ViewportPath takes a given path in order to traverse it, with the difference that all other traversing methods use the minimap to walk, while ViewportPath uses the coordinates in your viewport (aka 3d world) to walk.
This is pretty useful for accurate walking and a good way to make your bot act more humanlike.
This is pretty useful for accurate walking and a good way to make your bot act more humanlike.
Note that it is not required to use BresenhamPath, ViewportPath.convert(path)takes any kind of path.
Code:
public class Walking extends Task {
private final Coordinate bankCoord = new Coordinate(x, y);
@Override
public void execute() {
final BresenhamPath path = BresenhamPath.buildTo(bankCoord);
if (path != null) { // Although BresenhamPath technically always builds a path, it is recommended to nullcheck rather than having the bot crash
if (bankCoord.distanceTo(Players.getLocal()) >= 8 || !ViewportPath.convert(path).step()) { // this will attempt to walk with the viewport if the distance to the destination is < 8
path.step(); // if it cant walk with viewport (camera not correclty set for example), step() will return false,
// causing the bot to walk with the minimap
}
}
}
@Override
public boolean validate() {
//validate
}
}
You also can combine those methods to ensure a good traversal, for example, let the bot generate a path with RegionPath, and nullcheck it in order to see if it is valid. If it is, you can let it walk, if not, attempt to build a WebPath, or BresenhamPath as a last resort.
Last edited: